You are viewing the RapidMiner Developers documentation for version 8.0 - Check here for latest version
Creating Custom Configurators
Imagine that you want to create a RapidMiner extension that offers an operator for reading data from a CRM system. Your operator needs the information for accessing the CRM, such as a URL, a user name, or a password. One approach is to add text fields to the parameters of the operator and let the user type in the required information. Though this may seem convenient, it gets quite redundant if you want to use the same information in other RapidMiner processes or operators, since you would have to enter the information multiple times. Alternatively, you can define the CRM connection globally and let the user select which CRM to get data from.
This is a scenario where Configurators
come in handy.
A configurator globally manages items of a certain type globally and allows you to create, edit and delete them through a custom configuration dialog.
For this example, we will implement a configurator for CRM entries that allows us to automatically configure those entries using a dialog accessible through the Connections menu.
Moreover, a configurator can be used along with a drop-down list, which allows the user to easily select a connection via a parameter of your operator.
Usage
In order to implement your own configurator, you need to know the following classes:
- Configurable is an item that can be modified through a Configurator.
- Configurator instantiates and configures subclasses of Configurable.
- ConfigurationManager is used to register Configurators in RapidMiner.
- ParameterTypeConfigurable is a ParameterType that creates a drop-down list for configurators and can be used in the parameter settings of operators.
First, create a new class describing a single CRM connection entry, which implements the Configurable interface. Best practice is to extend AbstractConfigurable instead, because by doing so, you avoid dealing with parameter values. Then, you don't have to write code that deals with the actual configuration:
public class CRMConfigurable extends AbstractConfigurable {
@Override
public String getTypeId() {
return "CRM";
}
/** Actual business logic of this configurable */
public CRMConnection connect() {
String username = getParameter("user name");
String url = getParameter("URL");
URLConnection con = new URL(url).openConnection();
// ...
// do something with the connection
}
}
Next, we have to extend the AbstractConfigurator class.
Each configurator has a unique typeId, a String in order to identify the configurator in RapidMiner and an I18NBaseKey which will be used as the base key for retrieving localized information from the resource file.
Also, we want to add some ParameterTypes to our Configurator, because they specify how an entry can be edited through the configuration dialog.
In our example, we need Parameter Types describing the URL, the user name and password which should be used for the CRM connection.
For that matter, you would simply have to overwrite the getParameterTypes
method and add a new ParameterTypeString
, as shown in the following implementation:
public class CRMConfigurator extends AbstractConfigurator<CRMConfigurable> {
@Override
public Class<CRMConfigurable> getConfigurableClass() {
return CRMConfigurable.class;
}
@Override
public String getI18NBaseKey() {
return "crmconfig";
}
@Override
public List<ParameterType> getParameterTypes(ParameterHandler parameterHandler) {
List<ParameterType> parameters = new ArrayList<ParameterType>();
parameters.add(new ParameterTypeString("URL", "The URL to connect to", false));
parameters.add(new ParameterTypeString("Username", "The user name for the CRM", false));
parameters.add(new ParameterTypePassword("Password", "The password for the CRM"));
return parameters;
}
@Override
public String getTypeId() {
return "CRM";
}
}
In addition to the methods getTypeId
, getI18NBaseKey
and getParameterTypes
, you must also implement the method getConfigurableClass
, which simply returns the used Configurable implementation class (in this case, the class CRMConfigurable
).
Next, add localized information to the resource file GUIXXX.xml, where 'XXX' is the extension name.
gui.configurable.crmconfig.name = CRM Connection
gui.configurable.crmconfig.description = An entry describing a CRM connection.
gui.configurable.crmconfig.icon = data.png
To get access to the new configurator, register it in the ConfigurationManager. This step is important because it let's RapidMiner know of the new configurator so that the CRM operator and other parts of RapidMiner can access it. To do this, simply call the register method within the initialization procedure. This should be done in the initPlugin method of the PluginInit class:
public static void initPlugin() {
ConfigurationManager.getInstance().register(new CRMConfigurator());
}
You can now open the Manage Connections dialog in the Connections menu and create a CRM connection.
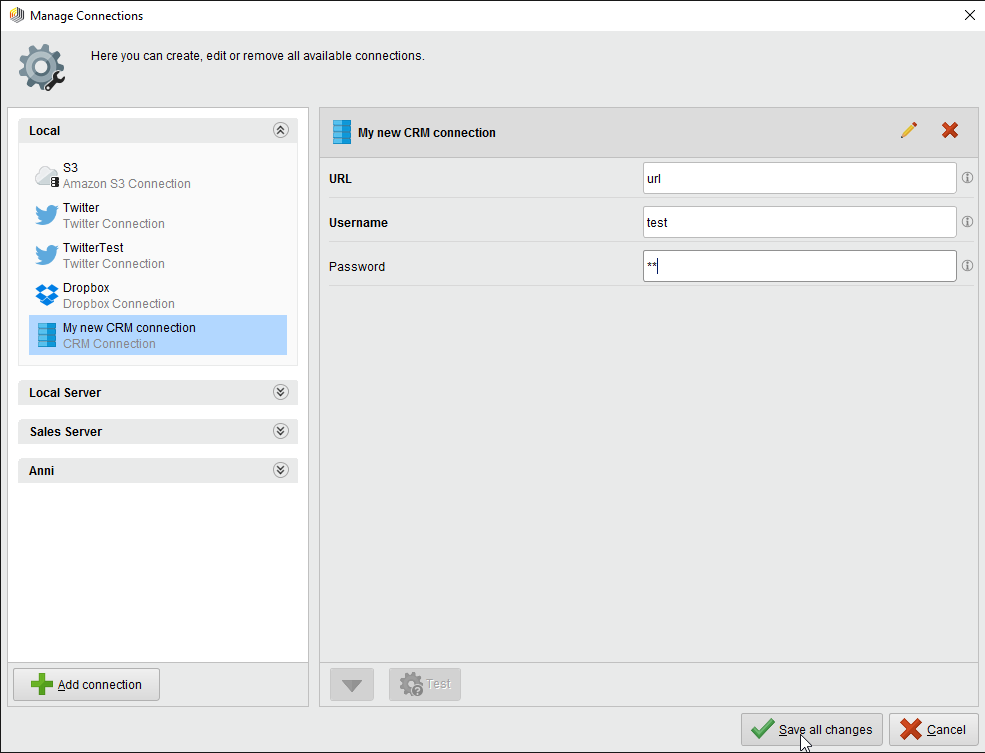
Now, use the connection as a parameter of an operator that connects to the CRM.
To do so, override the method getParameterTypes
and add ParameterTypeConfigurable
:
@Override
public List<ParameterType> getParameterTypes() {
List<ParameterType> types = super.getParameterTypes();
ParameterType type = new ParameterTypeConfigurable(PARAMETER_CONFIG, "Choose a CRM connection", "CRM");
types.add(type);
return types;
}
You have now successfully created your own configurator and can use it to configure CRM entries for your operator.
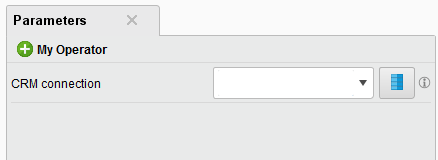